Hello Viewers in following example i'll show you how to send values using Intent.
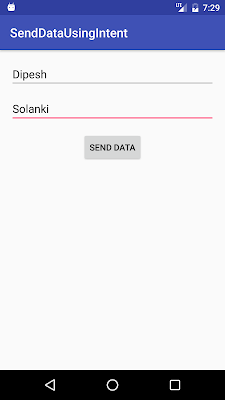
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.app.dipesh.senddatausingintent">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity android:name=".SecondActivity"></activity>
</application>
</manifest>
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
android:orientation="vertical"
tools:context="com.app.dipesh.senddatausingintent.MainActivity">
<EditText
android:id="@+id/efname"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Enter FirstName"/>
<EditText
android:id="@+id/elname"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Enter LastName"
android:layout_marginTop="10dp"/>
<Button
android:id="@+id/btnsend"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:text="Send Data"
android:layout_marginTop="15dp"/>
</LinearLayout>
ActivityMain.java
package com.app.dipesh.senddatausingintent;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class MainActivity extends AppCompatActivity {
EditText fname,lname;
Button sendData;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Find all views using findViewByid
fname = (EditText) findViewById(R.id.efname);
lname = (EditText) findViewById(R.id.elname);
sendData = (Button) findViewById(R.id.btnsend);
//set click listener on button.
sendData.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
//getting both edittext values.
String fnm = fname.getText().toString().trim();
String lnm = lname.getText().toString().trim();
Intent i = new Intent(getApplicationContext(),SecondActivity.class);
i.putExtra("fname",fnm);
i.putExtra("lname",lnm);
startActivity(i);
}
});
}
}
activity_second
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_second"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
android:orientation="vertical"
tools:context="com.app.dipesh.senddatausingintent.SecondActivity">
<TextView
android:id="@+id/tvfname"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"/>
<TextView
android:id="@+id/tvlname"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"/>
<Button
android:id="@+id/btnback"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Go Back"
android:layout_gravity="center_horizontal"
android:layout_marginTop="15dp"/>
</LinearLayout>
secondactivity.java
package com.app.dipesh.senddatausingintent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
public class SecondActivity extends AppCompatActivity {
TextView fname,lname;
Button back;
String fnm,lnm;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_second);
fname = (TextView) findViewById(R.id.tvfname);
lname = (TextView) findViewById(R.id.tvlname);
back = (Button) findViewById(R.id.btnback);
//check intent is null or not
if(!getIntent().getExtras().getString("fname").equals("") && !getIntent().getExtras().getString("lname").equals("")){
//get values from intent
fnm = getIntent().getExtras().getString("fname");
lnm = getIntent().getExtras().getString("lname");
fname.setText("First name : " + fnm);
lname.setText("Last name : " + lnm);
}else {
Toast.makeText(getApplicationContext(),"Intent is null",Toast.LENGTH_LONG).show();
}
back.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
finish();
}
});
}
}
No comments:
Post a Comment